Let's see how we can highlight a row in a CSS grid when we hover it. In this example, we will just change the background color of the hovered row, but you can replace this with any other effect.
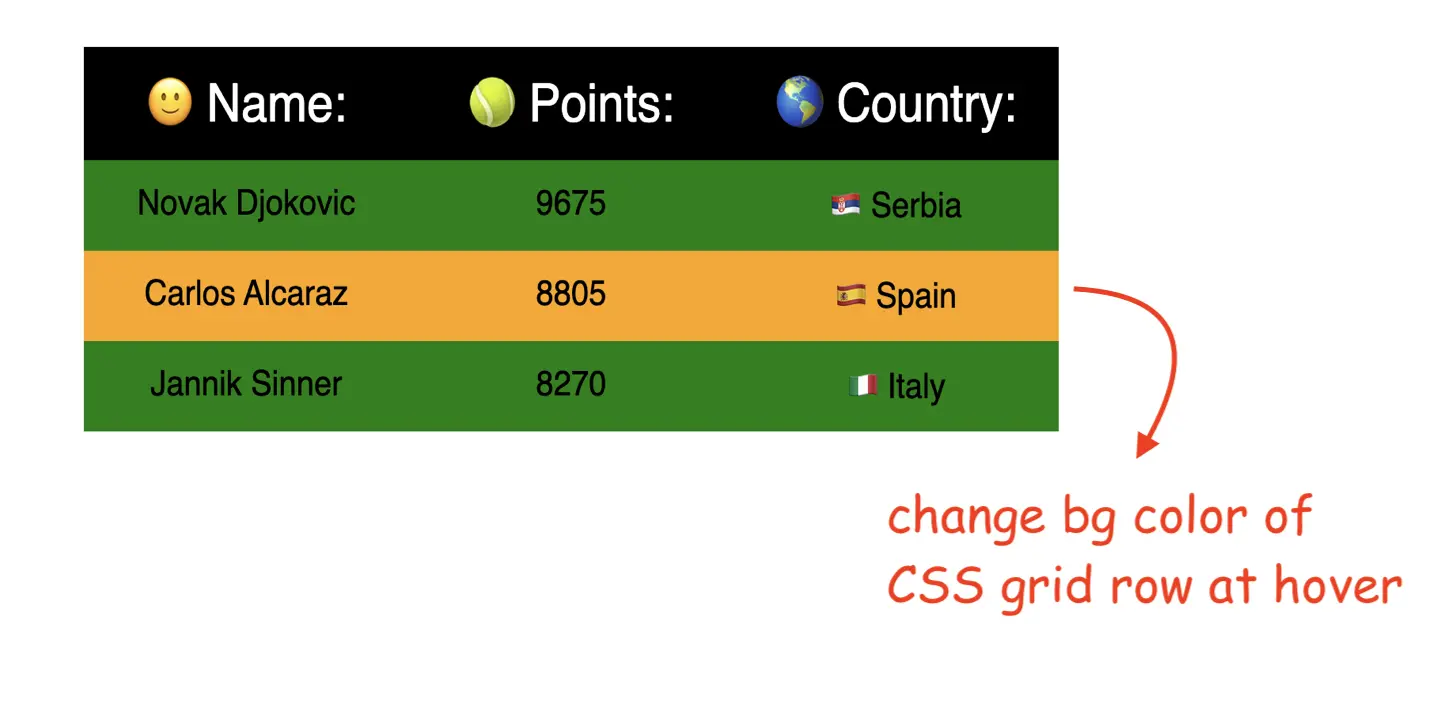
If you want to skip ahead the full code is on my Github and you can see the live example here.
Also If you want to watch this tutorial, this article is available also as a Youtube video. Take a look:
As great as CSS Grid is, there are some things it does not know how to do right of the box. One of these things is selecting rows or columns. We will need to add a small CSS hack to achieve this. Let's see how we can do it!
The initial setup of the Grid
Below we have the HTML for the data:
<div class="grid">
<div class="heading">š Name:</div>
<div class="heading">š¾ Points:</div>
<div class="heading">š Country:</div>
<div class="cell"><span>Novak Djokovic</span></div>
<div class="cell"><span>9675</span></div>
<div class="cell"><span>š·šø Serbia</span></div>
<div class="cell"><span>Carlos Alcaraz</span></div>
<div class="cell"><span>8805</span></div>
<div class="cell"><span>šŖšø Spain</span></div>
<div class="cell"><span>Jannik Sinner</span></div>
<div class="cell"><span>8270</span></div>
<div class="cell"><span>š®š¹ Italy</span></div>
</div>
And, this CSS will set setup the initial grid.
.grid {
display: grid;
grid-template-columns: 1fr 1fr 1fr;
background: green;
font-family: sans-serif;
}
.grid > * {
padding: 10px;
text-align: center;
}
.heading {
background: black;
color: #fff;
font-size: 150%;
}
If you don't need it, you can remove the header of the grid and the code will become a bit more simpler.
Using pseudo-elements to create a row hover effect in a CSS grid
As said we don't have an off-the-shelf solution for targeting a row, or column in a grid. CSS grids don't treat individual rows as separate entities.
But we can use a ::before
or ::after
element to simulate the row hover effect. These are the steps to achieve this:
- add a
::before
pseudo-element to all the data cells in Grid - using
position:absolute
we can make the pseudo-element expand to the full width of the row - change the background color of
::before
when we hover that cell and this will change also the color of the row
Below is the CSS that will generate the row hover effect:
.grid {
overflow: hidden;
}
.cell {
position: relative;
span {
position: relative;
z-index: 1;
}
&::before {
content: '';
position: absolute;
top: 0;
bottom: 0;
right: -1000%;
left: -1000%;
background-color: green;
}
&:hover::before {
background-color: orange;
}
}
Some things to notice here. We will need to use that extra span
inside of each cell to fix the z-index stacking context issue. By default the ::before
pseudo-element will overlap our content. So, doing a position relative to the span will break the stacking context, so we can place it on top of ::before
.
Also, note the overflow set to hidden for the grid to prevent the pseudo elements from going outside the main grid container.
You can see the full code here and the live example on Github pages.
By the way, if you found this article interesting you can also check out how to increase an element on hover in CSS grid. Happy coding!
š 50 Javascript, React and NextJs Projects
Learn by doing with this FREE ebook! Not sure what to build? Dive in with 50 projects with project briefs and wireframes! Choose from 8 project categories and get started right away.
š 50 Javascript, React and NextJs Projects
Learn by doing with this FREE ebook! Not sure what to build? Dive in with 50 projects with project briefs and wireframes! Choose from 8 project categories and get started right away.